Description
Turn data into functions! A simple and functional machine learning library written in elixir.
Emel alternatives and similar packages
Based on the "Statistics" category.
Alternatively, view Emel alternatives based on common mentions on social networks and blogs.
-
Plausible Analytics
Simple, open source, lightweight (< 1 KB) and privacy-friendly web analytics alternative to Google Analytics. -
numerix
A collection of useful mathematical functions in Elixir with a slant towards statistics, linear algebra and machine learning -
Statistex
Calculate statistics on data sets, reusing previously calculated values or just all metrics at once. Part of the benchee library family. -
simple_stat_ex
Simple Stat Ex can be used to keep counters around hourly daily or other activity for an elixir project
WorkOS - The modern identity platform for B2B SaaS
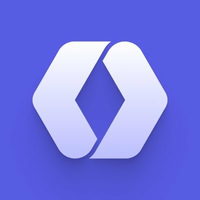
Do you think we are missing an alternative of Emel or a related project?
Popular Comparisons
README
# emel
Turn data into functions! A simple and functional machine learning library written in elixir.
## Installation
The package can be installed by adding emel
to your list of dependencies in mix.exs
:
def deps do
[
{:emel, "~> 0.3.0"}
]
end
The docs can be found at https://hexdocs.pm/emel/0.3.0.
## Usage
# set up the aliases for the module
alias Emel.Ml.KNearestNeighbors, as: KNN
dataset = [
%{"x1" => 0.0, "x2" => 0.0, "x3" => 0.0, "y" => 0.0},
%{"x1" => 0.5, "x2" => 0.5, "x3" => 0.5, "y" => 1.5},
%{"x1" => 1.0, "x2" => 1.0, "x3" => 1.0, "y" => 3.0},
%{"x1" => 1.5, "x2" => 1.5, "x3" => 1.5, "y" => 4.5},
%{"x1" => 2.0, "x2" => 2.0, "x3" => 2.0, "y" => 6.0},
%{"x1" => 2.5, "x2" => 2.5, "x3" => 2.5, "y" => 7.5},
%{"x1" => 3.0, "x2" => 3.3, "x3" => 3.0, "y" => 9.0}
]
# turn the dataset into a function
f = KNN.predictor(dataset, ["x1", "x2", "x3"], "y", 2)
# make predictions
f.(%{"x1" => 1.725, "x2" => 1.725, "x3" => 1.725})
# 5.25
### Implemented Algorithms
- Linear Regression
- K Nearest Neighbors
- Decision Tree
- Naive Bayes
- K Means
- Perceptron
- Logistic Regression
- Neural Network
alias Emel.Ml.DecisionTree, as: DecisionTree
alias Emel.Help.Model, as: Mdl
alias Emel.Math.Statistics, as: Stat
dataset = [
%{risk: "high", collateral: "none", income: "low", debt: "high", credit_history: "bad"},
%{risk: "high", collateral: "none", income: "moderate", debt: "high", credit_history: "unknown"},
%{risk: "moderate", collateral: "none", income: "moderate", debt: "low", credit_history: "unknown"},
%{risk: "high", collateral: "none", income: "low", debt: "low", credit_history: "unknown"},
%{risk: "low", collateral: "none", income: "high", debt: "low", credit_history: "unknown"},
%{risk: "low", collateral: "adequate", income: "high", debt: "low", credit_history: "unknown"},
%{risk: "high", collateral: "none", income: "low", debt: "low", credit_history: "bad"},
%{risk: "moderate", collateral: "adequate", income: "high", debt: "low", credit_history: "bad"},
%{risk: "low", collateral: "none", income: "high", debt: "low", credit_history: "good"},
%{risk: "low", collateral: "adequate", income: "high", debt: "high", credit_history: "good"},
%{risk: "high", collateral: "none", income: "low", debt: "high", credit_history: "good"},
%{risk: "moderate", collateral: "none", income: "moderate", debt: "high", credit_history: "good"},
%{risk: "low", collateral: "none", income: "high", debt: "high", credit_history: "good"},
%{risk: "high", collateral: "none", income: "moderate", debt: "high", credit_history: "bad"}
]
{training_set, test_set} = Mdl.training_and_test_sets(dataset, 0.75)
f = DecisionTree.classifier(training_set, [:collateral, :income, :debt, :credit_history], :risk)
predictions = Enum.map(test_set, fn row -> f.(row) end)
actual_values = Enum.map(test_set, fn %{risk: v} -> v end)
Stat.similarity(predictions, actual_values)
# 0.75
### Mathematics