Description
Ecto.Rut is a wrapper around Ecto.Repo methods that usually require you to pass the module as the subject and sometimes even require you do extra work before hand, (as in the case of Repo.insert/3) to perform operations on your database. Ecto.Rut tries to reduce code repetition by following the "Convention over Configuration" ideology.
ecto_rut alternatives and similar packages
Based on the "ORM and Datamapping" category.
Alternatively, view ecto_rut alternatives based on common mentions on social networks and blogs.
-
paper_trail
Track and record all the changes in your database with Ecto. Revert back to anytime in history. -
ecto_psql_extras
Ecto PostgreSQL database performance insights. Locks, index usage, buffer cache hit ratios, vacuum stats and more.
WorkOS - The modern identity platform for B2B SaaS
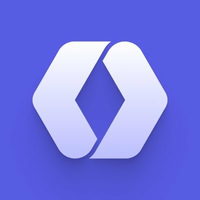
* Code Quality Rankings and insights are calculated and provided by Lumnify.
They vary from L1 to L5 with "L5" being the highest.
Do you think we are missing an alternative of ecto_rut or a related project?
README
Provides simple, sane and terse shortcuts for Ecto models.
Ecto.Rut is a wrapper around Ecto.Repo
methods that usually require you to pass
the module as the subject and sometimes even require you do extra work before hand,
(as in the case of Repo.insert/3
) to perform operations on your database. Ecto.Rut
tries to reduce code repetition by following the "Convention over Configuration"
ideology.
See the Ecto.Rut Documentation on HexDocs.
Basic Usage
You can call normal Ecto.Repo
methods directly on the Models:
Post.all
# instead of YourApp.Repo.all(Post)
Post.get(2)
# instead of YourApp.Repo.get(Post, 2)
Post.insert(title: "Awesome Post", slug: "awesome-post", category_id: 3)
# instead of:
# changeset = Post.changeset(%Post{}, %{title: "Awesome Post", slug: "awesome-post", category_id: 3})
# YourApp.Repo.insert(changeset)
See the Repo Coverage Section or the Hex Documentation for a full list of supported methods.
Installation
Add :ecto_rut
as a dependency in your mix.exs file:
defp deps do
[{:ecto_rut, "~> 1.2.2"}]
end
and run:
$ mix deps.get
Phoenix Projects
If you have an app built in Phoenix Framework, just add use Ecto.Rut
in the models
method in web/web.ex
or anywhere else you have defined your
basic Schema structure:
# web/web.ex
def model do
quote do
use Ecto.Schema
use Ecto.Rut, repo: YourApp.Repo
# Other stuff...
end
end
That's it! Ecto.Rut
will automatically be loaded in all of your models. If you don't
have a central macro defined for your Schema, take a look at this example:
Repo.Schema.
Other Ecto Projects
If you're not using Phoenix or you don't want to use Ecto.Rut with all of your models (why wouldn't
you!?), you'll have to manually add use Ecto.Rut
:
defmodule YourApp.Post do
use Ecto.Schema
use Ecto.Rut, repo: YourApp.Repo
# Schema, Changeset and other stuff...
end
Configuration
You do not need to configure Ecto.Rut unless your app is set up differently. All values are
inferred automatically and it should just work, but if you absolutely have to, you can specify
the repo
and model
modules:
defmodule YourApp.Post do
use Ecto.Schema
use Ecto.Rut, model: YourApp.Other.Post, repo: YourApp.Repo
end
See the Configuration Section in HexDocs for more details.
Not for Complex Queries
Ecto.Rut
has been designed for simple use cases. It's not meant for advanced queries and
operations on your Database. Ecto.Rut is supposed to do only simple tasks such as getting,
updating or deleting records. For any complex queries, you should use the original Ecto.Repo
methods.
You shouldn't also let Ecto.Rut handicap you; Ideally, you should understand how Ecto.Repo lets you do advanced queries and how Ecto.Rut places some limits on you (for example, not being able to specify custom options). JosΓ© has also shown his concern:
My concern with using something such as shortcuts is that people will forget those basic tenets schemas and repositories were built on.
That being said, Ecto.Rut's goal is to save time (and keystrokes) by following the convention
over configuration ideology. You shouldn't need to call YourApp.Repo
for every little task,
or involve yourself with changesets everytime when you've already defined them in your model.
Methods
All
iex> Post.all
[%Post{id: 1, title: "Post 1"},
%Post{id: 2, title: "Post 2"},
%Post{id: 3, title: "Post 3"}]
Get
iex> Post.get(2).title
"Blog Post No. 2"
Also see get!/1
Get By
Post.get_by(published_date: "2014-10-22")
Also see get_by!/1
Insert
You can insert Changesets, Structs, Keyword Lists or Maps. When Structs, Keyword Lists or Maps are given, they'll be first converted into a changeset (as defined by your model) and validated before inserting.
So you don't need to create a changeset every time before inserting a new record.
# Accepts Keyword Lists
Post.insert(title: "Introduction to Elixir")
# Accepts Maps
Post.insert(%{title: "Building your first Phoenix app"})
# Even accepts Structs (these would also be validated by your defined changeset)
Post.insert(%Post{title: "Concurrency in Elixir", categories: ["programming", "elixir"]})
Also see insert!/1
Update
There are two variations of the update method; update/1
and
update/2
. For the first, you can either pass a changeset (which would be
inserted immediately) or a modified struct (which would first be converted into a changeset
by comparing it with the existing record):
post = Post.get(2)
modified_post = %{ post | title: "New Post Title" }
Post.update(modified_post)
The second method is to pass the original record as the subject and a Keyword List (or a Map) of the new attributes. This method is recommended:
post = Post.get(2)
Post.update(post, title: "New Post Title")
Also see update!/1
and update!/2
Delete
post = Post.get_by(id: 9)
Post.delete(post)
Also see delete!/1
Delete All
Post.delete_all
Method Coverage
Ecto.Repo Methods | Ecto.Rut Methods | Additional Notes |
---|---|---|
Repo.aggregate | β | β |
Repo.all | Model.all | β |
Repo.config | β | β |
Repo.delete | Model.delete | β |
Repo.delete! | Model.delete! | β |
Repo.delete_all | Model.delete_all | β |
Repo.get | Model.get | β |
Repo.get! | Model.get! | β |
Repo.get_by | Model.get_by | β |
Repo.get_by! | Model.get_by! | β |
Repo.in_transaction? | β | β |
Repo.insert | Model.insert | Accepts structs, changesets, keyword lists and maps |
Repo.insert! | Model.insert! | Accepts structs, changesets, keyword lists and maps |
Repo.insert_all | β | β |
Repo.insert_or_update | β | β |
Repo.insert_or_update! | β | β |
Repo.one | β | β |
Repo.one! | β | β |
Repo.preload | β | β |
Repo.query | β | β |
Repo.query! | β | β |
Repo.rollback | β | β |
Repo.start_link | β | β |
Repo.stop | β | β |
Repo.transaction | β | β |
Repo.update | Model.update | Accepts structs, changesets, keyword lists and maps |
Repo.update! | Model.update! | Accepts structs, changesets, keyword lists and maps |
Repo.update_all | β | β |
Roadmap
- [x] Write Tests
- [x] Write Documentation
- [ ] Cover all main
Ecto.Repo
methods - [x] Allow explicitly passing Application and Repo modules to the
use Ecto.Rut
statement - [ ] Add support for preloading with existing methods
- [ ] Introduce new wrapper and helper methods that do not exist in Ecto, such as:
- [ ]
Model.count
- [ ]
Model.first
andModel.last
- [ ]
Model.find_or_create(obj)
- [ ] Methods that accept direct arguments (E.g.
delete_by_id(3)
)
- [ ]
Contributing
- Fork, Enhance, Send PR
- Lock issues with any bugs or feature requests
- Implement something from Roadmap
- Spread the word
License
This package is available as open source under the terms of the MIT License.
*Note that all licence references and agreements mentioned in the ecto_rut README section above
are relevant to that project's source code only.