Description
A library for interfacing with the Trello API.
ExTrello alternatives and similar packages
Based on the "Third Party APIs" category.
Alternatively, view ExTrello alternatives based on common mentions on social networks and blogs.
-
MongoosePush
MongoosePush is a simple Elixir RESTful service allowing to send push notification via FCM and/or APNS. -
cashier
Cashier is an Elixir library that aims to be an easy to use payment gateway, whilst offering the fault tolerance and scalability benefits of being built on top of Erlang/OTP -
airbrake
An Elixir notifier to the Airbrake/Errbit. System-wide error reporting enriched with the information from Plug and Phoenix channels.
InfluxDB - Power Real-Time Data Analytics at Scale
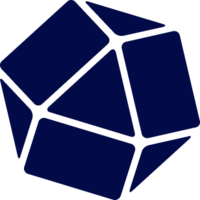
Do you think we are missing an alternative of ExTrello or a related project?
README
ExTrello 





A library for interfacing with the Trello API.
Heavily influenced by https://github.com/parroty/extwitter with some stuff straight ripped out of it.
Important! Migration from 0.x -> 1.x
Since this change will break all existing projects using ExTrello upon upgrading this deserves a spot at the top :)
All calls to the Trello API will now be wrapped in a tuple with the first element either being :ok
, :error
, or :connection_error
Regular errors will no longer raise exceptions as that is not idiomatic.
Here's an example(just a little sample):
# Old and bad
boards = ExTrello.boards()
# New and great
{:ok, boards} = ExTrello.boards()
# Old and bad, yuck!
try do
ExTrello.get("potato/2")
rescue
%ExTrello.Error{code: code, message: message} -> IO.puts "ERROR[#{code}] - #{message}"
end
# New and fantastic
case ExTrello.get("potato/2") do
{:ok, response} -> response
{:error, %ExTrello.Error{code: code, message: message}} -> IO.puts "ERROR[#{code}] - #{message}"
{:connection_error, %ExTrello.ConnectionError{reason: _, message: message}} -> IO.puts "#{message} We should retry."
end
Documentation
Installation
Add
ex_trello
to your list of dependencies inmix.exs
:def deps do [ ..., {:ex_trello, "~> 1.1.0"} ] end
Ensure
ex_trello
is started before your application:def application do [applications: [:ex_trello]] end
Run
mix deps.get
Usage
- Make sure you've completed installation.
- Use
ExTrello.configure
to setup Trello's OAuth authentication parameters. - Call functions in the
ExTrello
module. (e.g.ExTrello.boards()
)
Configuration
The default behavior is for ExTrello to use the application environment:
In config/<env>.exs
add:
# I like using ENV vars to populate my configuration. But fill this out however you'd like :)
config :ex_trello, :oauth, [
consumer_key: System.get_env("TRELLO_CONSUMER_KEY"),
consumer_secret: System.get_env("TRELLO_CONSUMER_SECRET"),
token: System.get_env("TRELLO_ACCESS_TOKEN"),
token_secret: System.get_env("TRELLO_ACCESS_SECRET")
]
You can also manually configure it at runtime:
ExTrello.configure(consumer_key: "...", ...)
You can also configure for the current process only:
defmodule TrelloServer do
use GenServer
def start_link(user) do
GenServer.start_link(__MODULE__, user, [])
end
def init(%User{token: token, token_secret: token_secret}) do
:ok = ExTrello.configure(
:process,
consumer_key: System.get_env("TRELLO_CONSUMER_KEY"),
consumer_secret: System.get_env("TRELLO_CONSUMER_SECRET"),
token: token,
token_secret: token_secret
)
{:ok, %{boards: []}}
end
# Rest of your code...
end
Samples
Authorize your application
Example for authorization. This is a naive solution that only works for demonstration. TODO: Set up example application.
# First we have to get a request token from Trello.
{:ok, token} = ExTrello.request_token("http://localhost:4000/auth/trello/callback/1234")
# We have to store this token because we need the `oauth_token_secret` after the callback to obtain our access token & secret.
# e.g. TokenAgent.store("1234", token.oauth_token_secret)
# Generate the url for authorization
{:ok, auth_url} = ExTrello.authorize_url(token.oauth_token, %{return_url: "http://localhost:4000/auth/trello/callback/1234", scope: "read,write", expiration: "never", name: "Your Application Name here"})
# Copy the url and visit it using your browser.
IO.puts auth_url
After signing in/authorizing the application you will be redirected to the callback URL you specified in the Request token & authorize URL.
Example:
http://localhost:4000/auth/trello/callback/1234?oauth_token=**copy_this**&oauth_verifier=**copy_this**
Copy the oauth_token
and oauth_verifier
above:
oauth_token = "copied_oauth_token"
oauth_verifier = "copied_oauth_verifier"
oauth_token_secret = retrieve_oauth_token_secret_from_before() # e.g. TokenAgent.retrieve("1234") from hypothetical TokenAgent
{:ok, access_token} = ExTrello.access_token(oauth_verifier, oauth_token, oauth_token_secret)
# Let's configure ExTrello with our newly obtained access token
ExTrello.configure(
consumer_key: System.get_env("TRELLO_CONSUMER_KEY"),
consumer_secret: System.get_env("TRELLO_CONSUMER_SECRET"),
token: access_token.oauth_token,
token_secret: access_token.oauth_token_secret
)
# Testing our token!
{:ok, member} = ExTrello.member() # Fetches the authenticated member record from Trello
TODO:
- [ ] Add models for
label,checklist,member, notification,organization, session, token,action - [ ] Pagination
- [ ] Code Cleanup! (Perhaps remove
defapicall
macro and use thewith
keyword instead? Have to evaluate.) - [ ] Example Application
- [ ] Investigate handling storage of request_token.oauth_token_secret instead of leaving that up to the dev.